Table Of Content
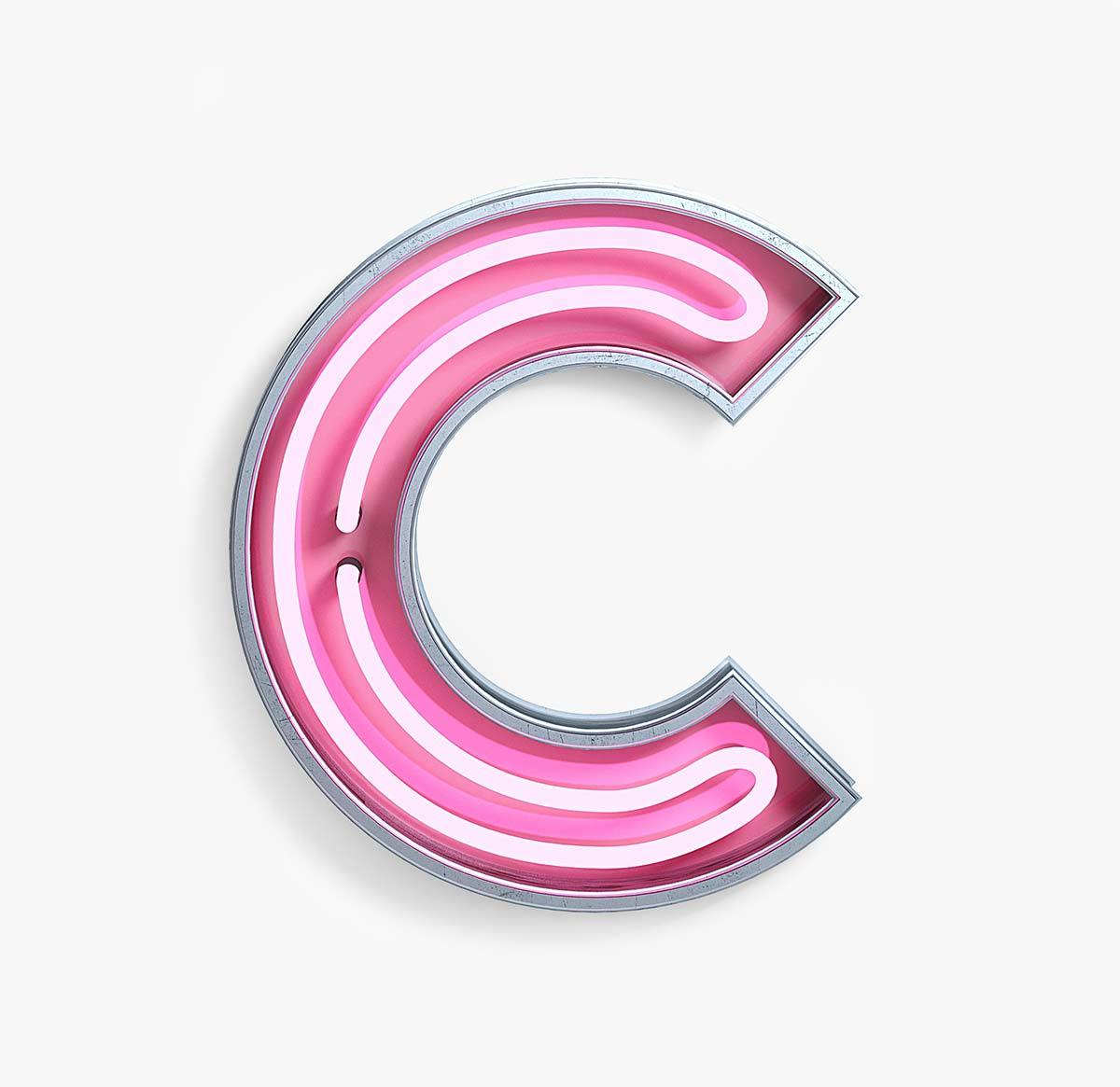
Instead of accessing the GPIO register directly, we now store a reference to that register in a global variable, and later dereference that variable, which will let us access the actual register again. By the time led_on() is executed, the function doesn’t care anymore which I/O pin the LED is actually connected to. Admittedly, this particular example won’t quite justify the added complexity, we can achieve more or less the same with preprocessor macros. However, say we wanted to control multiple LEDs connected arbitrarily, or have a generic handler for polling multiple inputs, we could now store the references in an array and loop through it.
C Language
(Although char can represent any of C's "basic" characters, a wider type may be required for international character sets.) Most integer types have both signed and unsigned varieties, designated by the signed and unsigned keywords. Signed integer types may use a two's complement, ones' complement, or sign-and-magnitude representation. In many cases, there are multiple equivalent ways to designate the type; for example, signed short int and short are synonymous.
Application programming interface (API)
A continue not contained within a nested iteration statement is the same as goto cont. The identifier must be a label (followed by a colon) located in the current function. A missing second expression makes the while test always non-zero, creating a potentially infinite loop. In the if statement, if the in parentheses is nonzero (true), control passes to . If the else clause is present and the is zero (false), control will pass to . The else part is optional and, if absent, a false will simply result in skipping over the .
Character set
It is designed to allow for programs that are extremely terse, have a close relationship with the resulting object code, and yet provide relatively high-level data abstraction. C was the first widely successful high-level language for portable operating-system development. C is sometimes used as an intermediate language by implementations of other languages. This approach may be used for portability or convenience; by using C as an intermediate language, additional machine-specific code generators are not necessary. C has some features, such as line-number preprocessor directives and optional superfluous commas at the end of initializer lists, that support compilation of generated code. However, some of C's shortcomings have prompted the development of other C-based languages specifically designed for use as intermediate languages, such as C--.
Strings
Some implementations are not hosted, usually because they are not intended to be used with an operating system. A free-standing implementation is free to specify how it handles program startup; in particular it need not require a program to define a main function. Assigning values to individual members of structures and unions is syntactically identical to assigning values to any other object. The only difference is that the lvalue of the assignment is the name of the member, as accessed by the syntax mentioned above. Because certain characters cannot be part of a literal string expression directly, they are instead identified by an escape sequence starting with a backslash (\).
Although the syntax for parameter declarations was augmented to include the style used in C++, the K&R interface continued to be permitted, for compatibility with existing source code. C's integer types come in different fixed sizes, capable of representing various ranges of numbers. The type char occupies exactly one byte (the smallest addressable storage unit), which is typically 8 bits wide.
Why a C-130 Crew Braved a 26 Hour Flight to Guam - Air & Space Forces Magazine
Why a C-130 Crew Braved a 26 Hour Flight to Guam.
Posted: Tue, 23 Apr 2024 22:30:00 GMT [source]
A common alternative to wchar_t is to use a variable-width encoding, whereby a logical character may extend over multiple positions of the string. Variable-width strings may be encoded into literals verbatim, at the risk of confusing the compiler, or using numerical backslash escapes (e.g. "\xc3\xa9" for "é" in UTF-8). The UTF-8 encoding was specifically designed (under Plan 9) for compatibility with the standard library string functions; supporting features of the encoding include a lack of embedded nulls, no valid interpretations for subsequences, and trivial resynchronisation.
Also, bit field types specified as plain int may be signed or unsigned, depending on the compiler. C enables programmers to create efficient implementations of algorithms and data structures, because the layer of abstraction from hardware is thin, and its overhead is low, an important criterion for computationally intensive programs. For example, the GNU Multiple Precision Arithmetic Library, the GNU Scientific Library, Mathematica, and MATLAB are completely or partially written in C. Many languages support calling library functions in C, for example, the Python-based framework NumPy uses C for the high-performance and hardware-interacting aspects.
By the beginning of the 1980s compatibility problems between the various C implementations became apparent. In 1983 the American National Standards Institute (ANSI) formed a committee to establish a standard specification of C known as "ANSI C". This work culminated in the creation of the so-called C89 standard in 1989. Part of the resulting standard was a set of software libraries called the ANSI C standard library. Compiled applications written in C are either statically linked with a C library, or linked to a dynamic version of the library that is shipped with these applications, rather than relied upon to be present on the targeted systems. Functions in a compiler's C library are not regarded as interfaces to Microsoft Windows.
When used inside a block, it indicates that the storage has been defined by a declaration outside of that block. When used outside of all blocks, it indicates that the storage has been defined outside of the compilation unit. The extern storage class specifier is redundant when used on a function declaration. It indicates that the declared function has been defined outside of the compilation unit. A number of tools have been developed to help C programmers find and fix statements with undefined behavior or possibly erroneous expressions, with greater rigor than that provided by the compiler.
Structures (and pointers to structures) may also be used as function parameter and return types. Members of structures and unions cannot have an incomplete or function type. Thus members cannot be an instance of the structure or union being declared (because it is incomplete at that point) but can be pointers to the type being declared. The now generally recommended method[note 3] of supporting international characters is through UTF-8, which is stored in char arrays, and can be written directly in the source code if using a UTF-8 editor, because UTF-8 is a direct ASCII extension. Like string literals, character constants can also be modified by prefixes, for example L'A' has type wchar_t and represents the character value of "A" in the wide character encoding.
This declares the enum colors type; the int constants RED (whose value is 0), GREEN (whose value is one greater than RED, 1), BLUE (whose value is the given value, 5), and YELLOW (whose value is one greater than BLUE, 6); and the enum colors variable paint_color. The constants may be used outside of the context of the enum (where any integer value is allowed), and values other than the constants may be assigned to paint_color, or any other variable of type enum colors. C's usual arithmetic conversions allow for efficient code to be generated, but can sometimes produce unexpected results. For example, a comparison of signed and unsigned integers of equal width requires a conversion of the signed value to unsigned. In 2008, the C Standards Committee published a technical report extending the C language[26] to address these issues by providing a common standard for all implementations to adhere to.
The POSIX standard added several nonstandard C headers for Unix-specific functionality. A number of other groups are using other nonstandard headers – the GNU C Library has alloca.h, and OpenVMS has the va_count() function. This is a perfectly valid cast, and as long as accessing members of bar won’t go beyond the size of foo, we will be on the safe side. Whether such a type cast makes sense or not is of course a different story, and depends on the context, but pointers give us the freedom to cast as we please. Another way to use this freedom is assembling sequential data to one common buffer.
A character constant cannot be empty (i.e. '' is invalid syntax), although a string may be (it still has the null terminating character). Multi-character constants (e.g. 'xy') are valid, although rarely useful — they let one store several characters in an integer (e.g. 4 ASCII characters can fit in a 32-bit integer, 8 in a 64-bit one). Since the order in which the characters are packed into an int is not specified (left to the implementation to define), portable use of multi-character constants is difficult. A multidimensional array should not be confused with an array of pointers to arrays (also known as an Iliffe vector or sometimes an array of arrays). The former is always rectangular (all subarrays must be the same size), and occupies a contiguous region of memory. The latter is a one-dimensional array of pointers, each of which may point to the first element of a subarray in a different place in memory, and the sub-arrays do not have to be the same size.
At the same time, C rules for the use of arrays in expressions cause the value of a in the call to setArray to be converted to a pointer to the first element of array a. Thus, in fact this is still an example of pass-by-value, with the caveat that it is the address of the first element of the array being passed by value, not the contents of the array. And are used as the body of a function or anywhere that a single statement is expected.
No comments:
Post a Comment